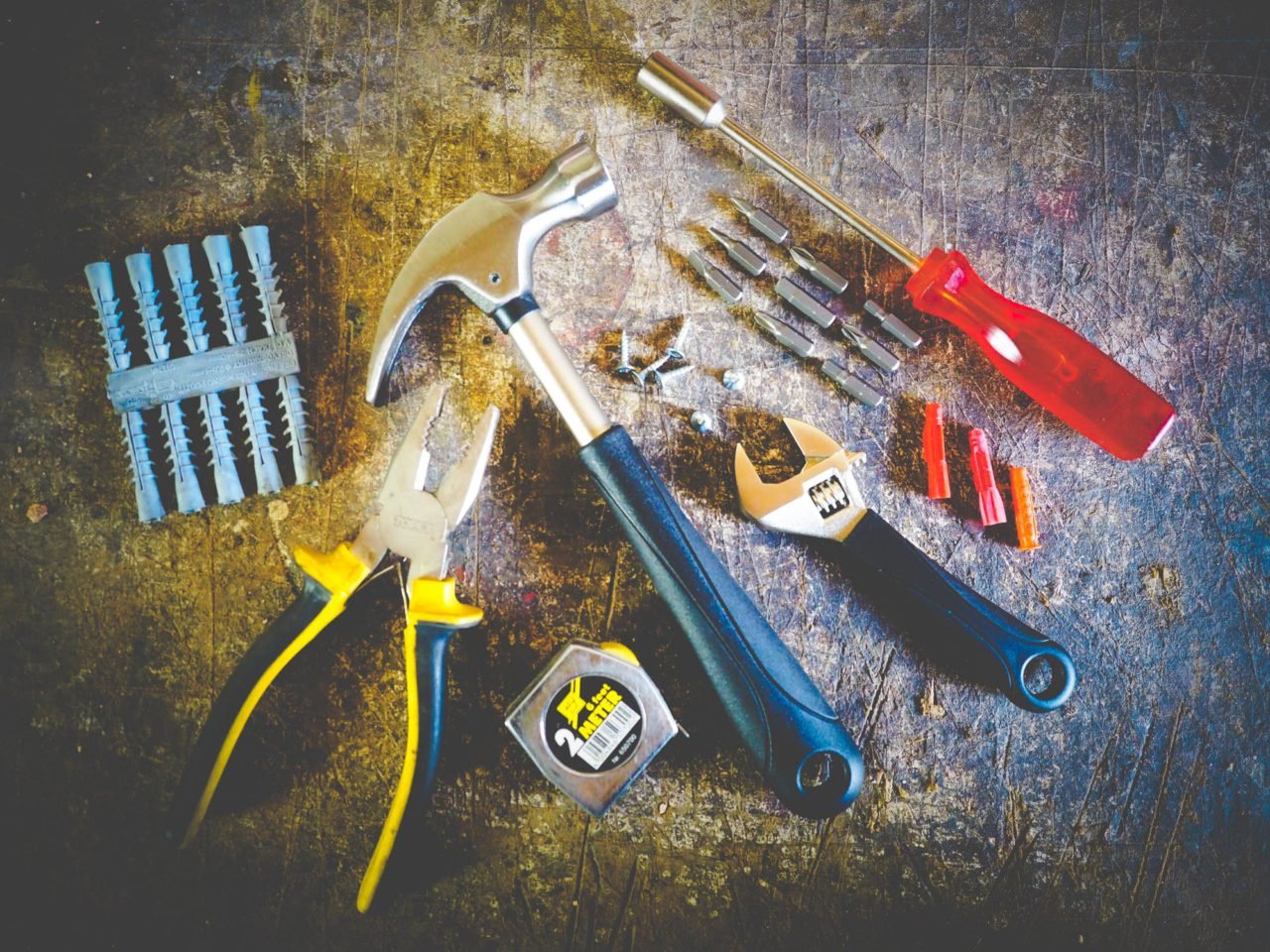
For those who are new to Python development, it is good to have a roadmap of what tools are needed to get started with development. For those who are more experienced with development, it is nice to have all the links in one place so you don’t have to go searching everytime.
This article lists the tools that are needed to get going with python development and a simple guide for getting all the tools installed
Launch Terminal
The terminal is the CLI interface for MacOS. It is similar to the CLI interface on unix/linux since MacOS is based off BSD unix. It is also similar to the Command prompt or powershell interface on Windows.
To get to the MacOS terminal:
- Click on the Go Menu, Select Utilities
- Double click on Terminal
I recommend pinning the terminal application to your dock.
Install XCode CLI tools
XCode is the development tool that Apple ships with MacOS. If you wanted to develop iPhone apps, etc… you would do it with XCode. XCode is not strictly required for python development. But when you install xcode, it installs the dependencies for Homebrew (See next section) and git version control which you will want to use is if you start collaborating with others on your projects.
To install XCode CLI tools, you have two options: Install it via the CLI, or through XCode.
To install via the CLI:
- Go back to your terminal window and run:
xcode-select --install
- Click yes on any prompts that appear
To install via the GUI:
- Open the app store, search for XCode
- Install XCode
- Launch XCode
- Click on the XCode Menu, Select Preferences
- Click on the Downloads tab
- Click on Components
- Click install next to command line tools
- Follow any prompts that appear
Install Homebrew
Home brew is a package manager for MacOS. It is similar to Apt-Get for those who have used Ubunut, or Yum for those who have used redhat linux. Package managers let you install programs on your computer from your terminal without having to go hunting for where to get the installation package.
To install homebrew, open your terminal window, and run the following command:
/bin/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)"
- Enter your password when prompted, then press return
- Press return again when prompted
Once installation completes, you will be ready to install apps.
Homebrew has two package types:
- Brew
- Cask
The main difference is applications with a GUI will usually be a cask package. Others will be a brew package.
A couple examples:
- To install Firefox (Gui Application) : brew install –cask firefox
- to install nmap (non-gui): brew install nmap
Go head and try out one of the above examples to make sure homebrew is working. If you want to find the homebrew for an application, you can search the homebrew website here: https://formulae.brew.sh/
Install VS Code
VS Code is one of the most popular development environments in the world right now. It is written in Javascript and runs as an electron app. This means it runs on pretty much every platform.
Now that you are familar with homebew, the easy way to install VS Code is with homebrew by running:
brew install --cask visual-studio-code
You can also download vscode from here: https://code.visualstudio.com/download
Install Python
MacOS ships with python version 2.7 pre-installed. So you could skip this step if you only plan on doing python version 2 development. However, Python version 2 is no longer supported as of January 1, 2020. For this reason, you should install python3:
brew install python
To manually install python, go to: https://www.python.org/downloads/
Install virtual environments
Virtual environments allow you to install python packages for a specific project instead of for the entire system. This becomes more impportant as you do more development because the more development you do, the more likely it is that you will run into a conflict. You can find a whole article on virtual environments here.
To install virtual environments, we will use the pip utility. Pip is short for “Preferred isntaller program”. As mentioned earlier, you should be using python version 3. Assuch, you should also be using pip3. If you run the pip command on Macos, it will install python 2 packages. If you use the pip3 command, it will install python3 packages.
To install virtualenv, run the following
pip3 install virtualenv --user
You now have the virtual environments package installed and you are ready to get going with your python development.
Testing out your development environment
Now that we have all our components installed, you are ready to get started. Open finder, click applications and launch Visual Studio Code
Click on the file menu, select New File
In the text window that opened, type:
print('Hello World!')
Click File -> Save
Save the file as hello.py
Click on the terminal menu, select new terminal, then type the following:
python3 hello.py
Your terminal window should say “Hello World!”
Congratulations, you just ran your first python program. Your development environment is now working
Summary
You are now ready to get started with developing your python code. In case you come back and want everything in once place, the code block below contains all the commands in order so you can copy/paste them into your terminal window all in one shot.
xcode-select --install
/bin/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)"
brew install --cask visual-studio-code
brew install python
pip3 install virtualenv --user