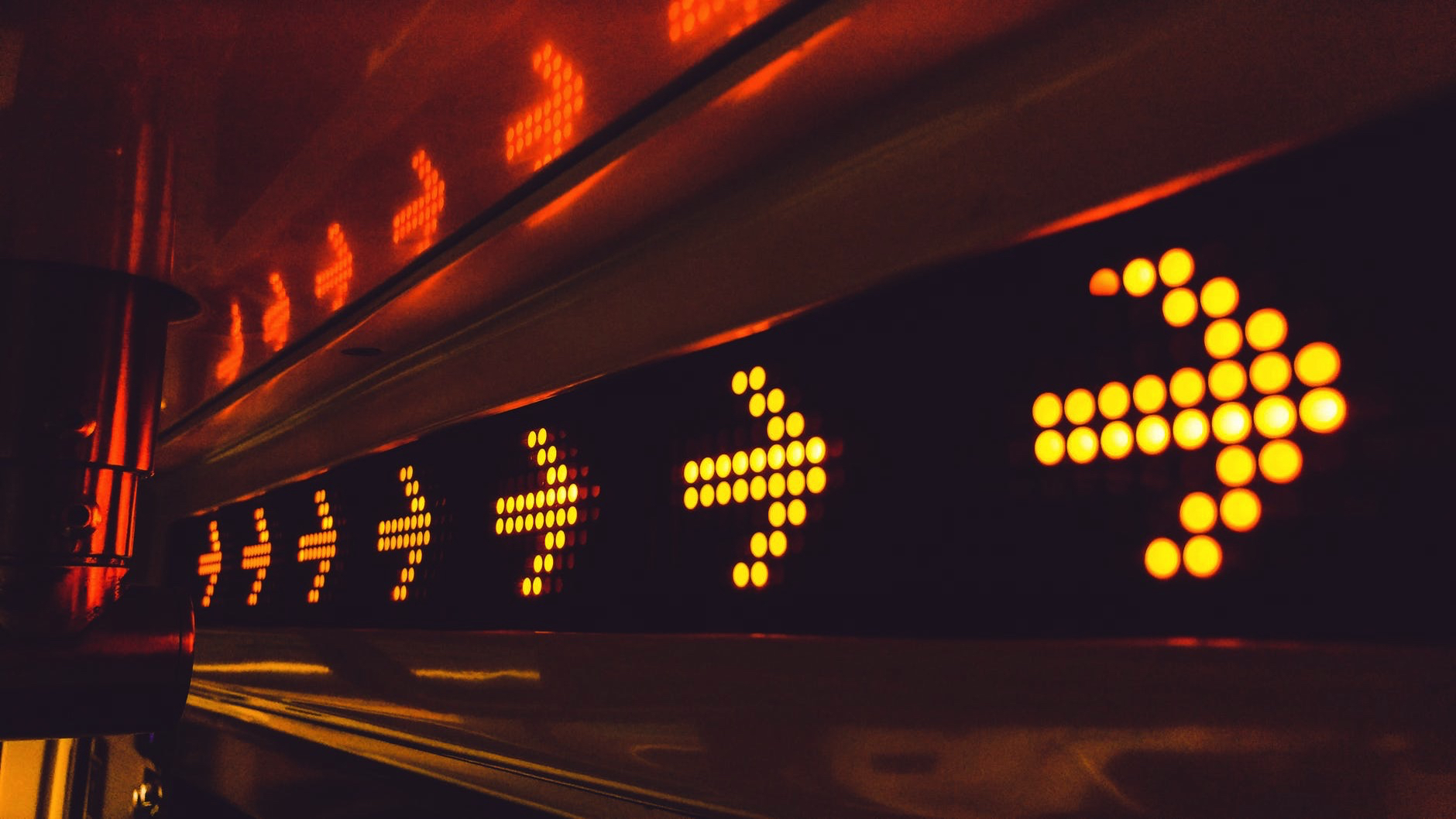
In languages like C, you have Switch statements allowing you to evaluate the input, then have a few options for the output. Switch statements are used in place of a series of if/else statements in order to make your code more readable. Case statements can also have better performance than a traditional if/else setup. This is because if you have a bunch of if statements, the system will have to evaluate all of the if statements at the time of execution. However, with a case statement, it is able to break out of that sequence once it finds a case that matches the input.
In C, a switch statement would look like this:
switch( key ) { case 'a' : result = 1; break; case 'b' : result = 2; break; default : result = -1; }
In the above code, you can see that you feed in the variable key. The system then evaluates the value of key and matches it up with the appropriate case. The system then runs the code within that code block.
Unfortunately, python does not have a switch statement like the one above, but there are some alternatives as shown below.
Series of If/Else If statements
You can use a series of If/Else If statements to get something that looks something like a case statement. The down side to this approach is it runs in series starting with the if statement at the top, then works its way down to the bottom. That means it will take longer to run as you add more else if statements. And the one at the bottom of the list will run the slowest since it has to evaluate all of the other if statements above it.
x='b' if x=='a': print "1" elif x =='b': print "2" elif x =='c': print "3" else: print "default"
Dictionary Lookup
The dictionary lookup below gives you the performance of a case statement. However, it does not look quite the same as a case statement. In this case, we have an array with key/value pairs. We set key to a value, then we do a lookup in the array for the value equal to the key we passed. This is better than the if/else if statements because you only have to evaluate the key value once vs checking it over and over again.
key = 'b' Options = {'a': 1, 'b': 2,'c': 3} result = Options.get(key, 'default') print result
The above code proves a point. But most likely, you will want to do something more than simply return the value in a key/value pair. The code below shows how you can use the dictionary idea to call functions
def hello(): return "Hello" def goodbye(): return "Goodbye" def default(): return "Default" key = 'a' result = { 'a': hello(), 'b': goodbye() }.get(key, default()) print result
In the above code, we pass a value to key, then pass key to our dictionary.
- If key=a, it will say hello.
- If key=b, it will say goodbye.
- If key=something else, it will say default
Conclusion
As you can see, the dictionary lookup is more concise. But the If/Else If statements are a little more similar to the traditional case statement. There are yet more options such as writing your own function for handling the logic. However, I found that these two options were the easiest to understand.
If you are concerned about readability, the if/else if statements are probably better. If you are concerned about performance, the dictionary approach is probably better.