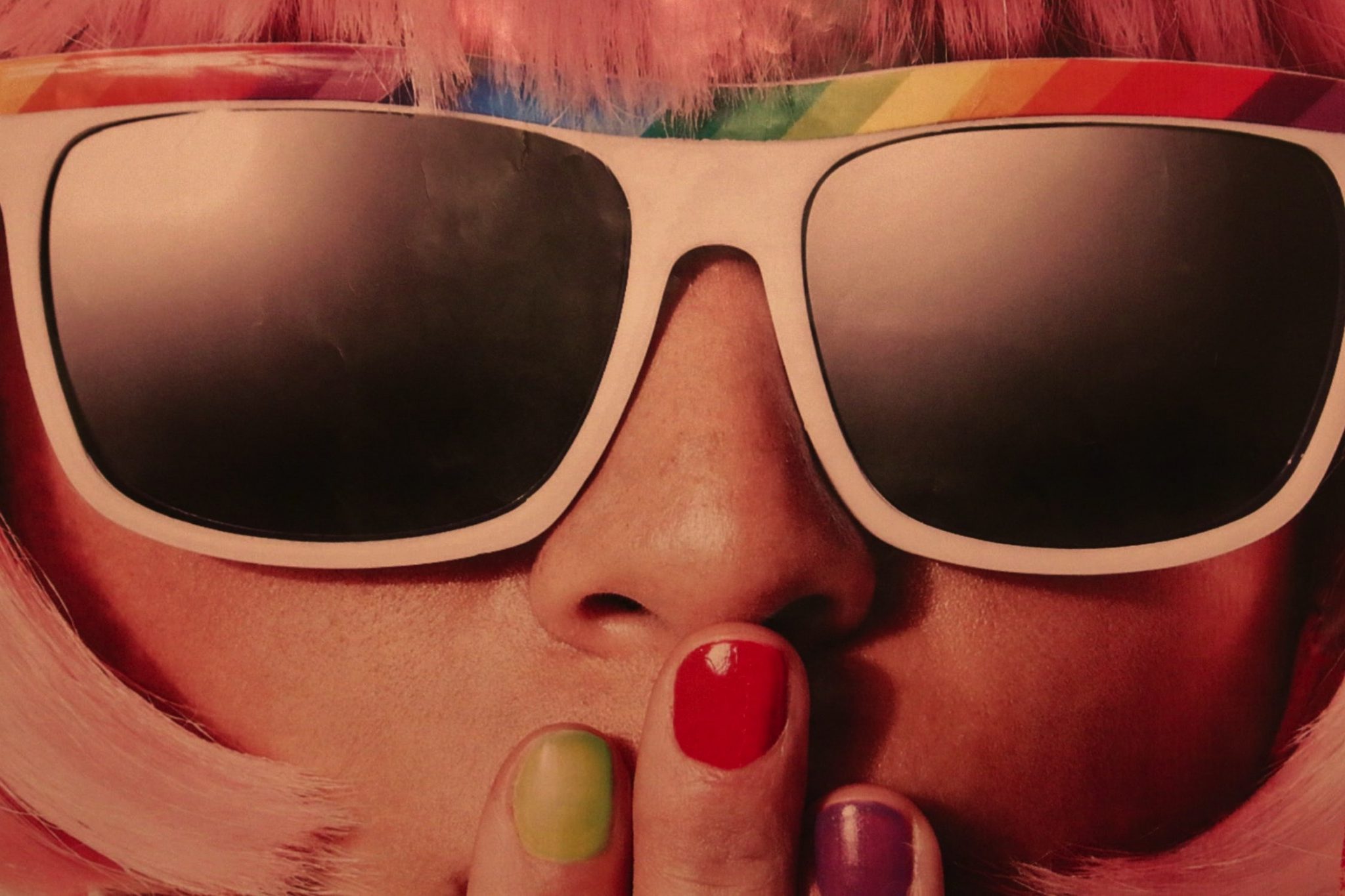
For those who want to do object or face detection, the standard way to do it is with OpenCV. OpenCV is a programming library for Object recognition and is very flexible. You can train it to recognize any number of different objects. For the purpose of this tutorial, I will be using Python3. However, you can use other programming languages with OpenCV as well.
Prerequisites
1. Pip
Pip is not necessarily a prerequisite. But it makes it a lot easier. Below are instructions on how to install Pip on OSX, Linux, and Windows.
Linux and OSX:
Open a terminal window and run: curl https://bootstrap.pypa.io/get-pip.py -o get-pip.py
Windows:
Installing on Windows is a very similar process to OSX and Linux, you just don’t have access to curl. So start by downloading the get-pip.py script here: https://bootstrap.pypa.io/get-pip.pyThen run:python3 https://bootstrap.pypa.io/get-pip.py
2. Opencv
If you are using python 3:
Pip3 install opencv-python
If you are using Python 2:
Pip install opencv-python
The above command(s) will install the latest version of OpenCV. As of today, that is version 3.4.
3. Pre-trained Model
OpenCV comes with pre-trained models for various scenarios. Using a pre-trained model is much easier than training one yourself. For the purposes of this demonstration, we will be using this pre-trained model.
There are additional pre-trained models here.
Running the script
After your prerequisites are installed, you can write your code. At the bottom of this article is an example script that will capture from your webcam and draw a rectangle around any detected faces. Make sure that you save the XML file you downloaded in the previous step to the same folder as the python script below.
The XML file I mentioned will detect all full faces. However, there are a variety of other pre-trained models you can use. There is one that will detect partial faces, another that will detect smiles, etc…. You can use the same script below with the different models to detect different things. In a future article, we will go over training your own model.
Below is the script I mentioned. You can copy and paste it into a file called face.py. Then you can run it my typing python3 face.py
######## Example ############## import cv2 import sys cascadePath = "haarcascade_frontalface_default.xml" faceCascade = cv2.CascadeClassifier(cascadePath) cam = cv2.VideoCapture(0) anterior = 0 while True: # Process each frame ret, frame = cam.read() gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY) faces = faceCascade.detectMultiScale( gray, scaleFactor=1.1, minNeighbors=5, minSize=(40, 40)) # Draw a rectangle around the faces for (x, y, w, h) in faces: cv2.rectangle(frame, (x, y), (x+w, y+h), (0, 255, 255), 2) if anterior != len(faces): anterior = len(faces) cv2.imshow('Video', frame) #Press Q to quit if cv2.waitKey(1) & 0xFF == ord('q'): break # Display video cv2.imshow('Video', frame) cam.release() cv2.destroyAllWindows()
When you are done running the above script, you can exit by pressing the ‘Q’ button on your keyboard.