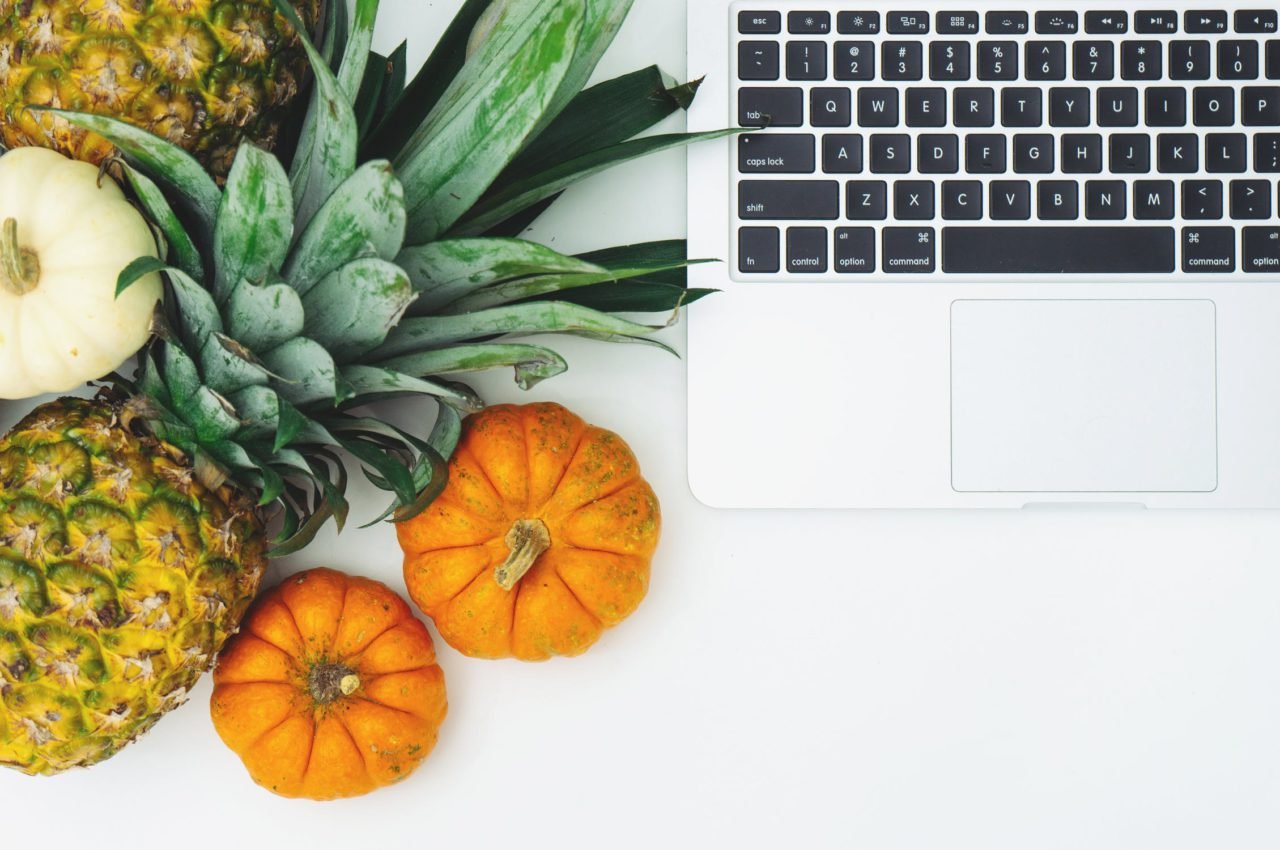
In python, almost everything is an object. This includes variables like String and Integers and containers like lists and dictionaries. Given that everything is an object, what are data types? Data types are simply how you classify your objects. If you have an object with the type of String, then that object will have all of the string properties and functions. If you have an object with a type of integer, then that object will have all the applicable properties and functions associated with integers.
Given most/all python of the data types, you will be working with are objects, there really is an infinite number of possible data types in Python. Any time you create a new class you are creating a new data type that can be used in new and unique ways. That said, there are some primary data types you will work with when you are just getting started. I have summarized what I think are the most common data types you need to look into.
Strings and characters
Strings and characters are two different data types. But they are somewhat related. In C++ a string is simply an array of characters. They are handled differently in python. But conceptually you can still think of them that way when you are doing string manipulation.
To keep things simple, you can think of a character as a string with a single number, letter, or special character such as ‘!’ or ‘@’ or ‘#’. A string is a bunch of characters to together which may or may not form readable words and sentences.
For information on how to use strings in Python, take a look here forĀ our article on using strings in Python.
Numbers
You have been using numbers since before you were in kindergarten, so you know what numbers are. And just like when you were in school, you started off with basic whole numbers, and things expanded from there.
There are four main types of numbers in Python:
- Integer
- Long Integer
- Float
- Complex
There are different scenarios when you will use the different number types in Python. But the main two types you will use are Integers and Floats. The difference being does the number have a decimal point. If yes, it is a float. If no, it is an integer. For more information on Python Numbers, look here. We talk about the four main kinds of numbers in Python, how to use them, and how the behavior of the different types vary.
Collections
Collections are the data type you use when you want to store something. There are four main kinds of collections in Python:
- List
- Dictionary
- Tuple
- Set
The most common collection type I use is a list. But there are plenty of scenarios to use the other four collections as well. As I said these are the four main types. There are many other kinds of collections in Python such as Chain maps, deque, counter, etc… These collection types are just not used quite as much as the other data types.
In general, a collection is a bunch of different objects stuck together. You collect your objects into a bucket, store everything in that bucket until you need to retrieve it. This bucket can store any kind of object, As an example, a list could store a string, an integer, and even another list. You can nest as many lists inside one another as you want, You just need to make sure you do things in a logical way.
For more information on Python collections, look here
Date time
Date time data types as you may have guessed are used for dates and times. You can store your dates and times as a string. However, doing things that way would require you to implement your own logic when you want to manipulate things.
As an example, you might have a date of 1/1/2019 and you wish to change the format. That is easy if you are using a Date Time data type. However, if you stored your date as a plain string, you now have to parse the string, figure out what the t\month, day, and year are, then implement your own logic on how to reformat the string. With a Date Time object, all of that work is already done for you.
Another example is if you want to add 1 day to the date. Instead of the value being 1/1/2019 you want the date to be 1/2/2019. Again, if you used a string, you have to implement your own logic regarding how to parse the string, increment only the middle number by 1. and then set your new string equal to the new value. By using the date time object, all of that logic is already written for you.
For more information on Python Date time, look here
Summary
Today we have discussed the four main data types in Python as well as what a data type is. As you learn more about Python, you will create your own data types. But these four data types are your foundation to build from.