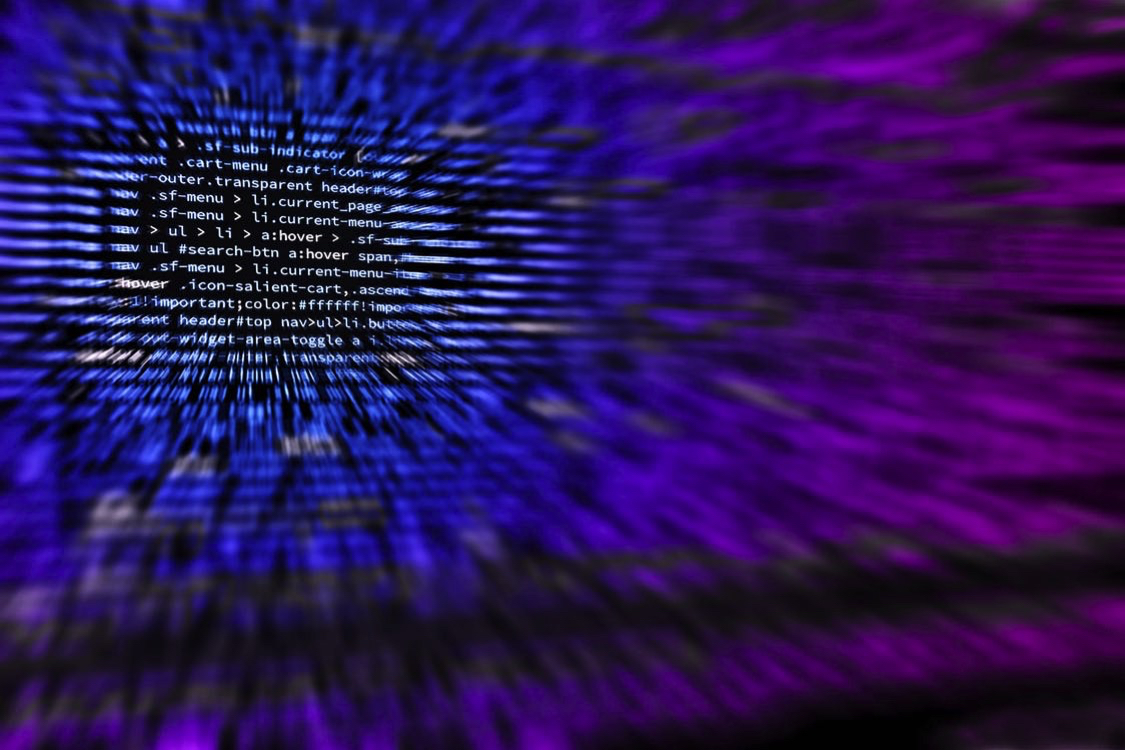
A common task in any programming language is Reading and Writing files. Below are a couple of functions that can be used for these purposes, along with descriptions of their use. Be sure to import java.io for these functions to work.
//Function for Reading Files. You need to pass a string with the path to the file you wish to read. This function will return a string value with the complete contents of the file in question.
static String readFile(String myFile){ String returnValue=""; try(BufferedReader br = new BufferedReader(new FileReader(myFile))) { for(String line; (line = br.readLine()) != null; ) { returnValue = returnValue + line + "\r\n"; } } catch(Exception e){} return returnValue; }
//Function for Writing files. You need to pass the string you wish to write to a file, along with the path to the file you wish to write to. This function will write the string to the file path you passed to it. This function will over-write any file that is already at that path.
static void writeFile(String myString, String myPath){ try{ PrintWriter writer = new PrintWriter(myPath, "UTF-8"); writer.print(myString); writer.close(); } catch(Exception e){} }